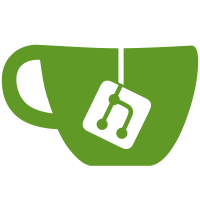
5 changed files with 84 additions and 52 deletions
@ -1,22 +0,0 @@ |
|||||||
<template> |
|
||||||
<div> |
|
||||||
<svg width="500" height="300"></svg> |
|
||||||
</div> |
|
||||||
</template> |
|
||||||
|
|
||||||
<script> |
|
||||||
const d3 = require('d3'); |
|
||||||
export default { |
|
||||||
mounted: function() { |
|
||||||
var svg = d3.select(this.$el).select('svg'); |
|
||||||
svg |
|
||||||
.append('circle') |
|
||||||
.attr('cx', '250') |
|
||||||
.attr('cy', '150') |
|
||||||
.attr('r', '100') |
|
||||||
} |
|
||||||
} |
|
||||||
</script> |
|
||||||
|
|
||||||
<style> |
|
||||||
</style> |
|
@ -1,25 +0,0 @@ |
|||||||
<!-- <template> |
|
||||||
Is should be missing, otherwise |
|
||||||
render() function will not execute |
|
||||||
</template> --> |
|
||||||
|
|
||||||
<script> |
|
||||||
const d3 = require('d3'); |
|
||||||
export default { |
|
||||||
render: function(createElement) { |
|
||||||
var el = createElement('svg') |
|
||||||
// console.log(el.elm); |
|
||||||
var svg = d3.select(el.elm); |
|
||||||
svg.attr('width', '500') |
|
||||||
// d3.select(el.elm) |
|
||||||
// .append('circle') |
|
||||||
// .attr('cx', '250') |
|
||||||
// .attr('cy', '150') |
|
||||||
// .attr('r', '100') |
|
||||||
return el; |
|
||||||
} |
|
||||||
} |
|
||||||
</script> |
|
||||||
|
|
||||||
<style> |
|
||||||
</style> |
|
@ -0,0 +1,30 @@ |
|||||||
|
<!-- |
||||||
|
Links: |
||||||
|
Components: https://vuejs.org/v2/guide/components.html |
||||||
|
|
||||||
|
Advanced: |
||||||
|
Component Lifecyrcle: https://vuejs.org/v2/guide/instance.html#Lifecycle-Diagram |
||||||
|
Virtaul DOM: https://medium.com/js-dojo/whats-new-in-vue-js-2-0-virtual-dom-dc4b5b827f40#.hexwxh9m3 |
||||||
|
--> |
||||||
|
|
||||||
|
<template> |
||||||
|
<svg width="500" height="300"></svg> |
||||||
|
</template> |
||||||
|
|
||||||
|
<script> |
||||||
|
const d3 = require('d3'); |
||||||
|
export default { |
||||||
|
mounted: function() { |
||||||
|
// this.#el - is the root element in <template> |
||||||
|
// in this case it is <svg> tag |
||||||
|
d3.select(this.$el) |
||||||
|
.append('circle') |
||||||
|
.attr('cx', '250') |
||||||
|
.attr('cy', '150') |
||||||
|
.attr('r', '100') |
||||||
|
} |
||||||
|
} |
||||||
|
</script> |
||||||
|
|
||||||
|
<style> |
||||||
|
</style> |
@ -0,0 +1,49 @@ |
|||||||
|
<!-- |
||||||
|
Links |
||||||
|
Data: https://vuejs.org/v2/guide/components.html#data-Must-Be-a-Function |
||||||
|
Form Input Bindings: https://vuejs.org/v2/guide/forms.html#Basic-Usage |
||||||
|
Watchers: https://vuejs.org/v2/guide/computed.html#Watchers |
||||||
|
--> |
||||||
|
|
||||||
|
<template> |
||||||
|
<div> |
||||||
|
<svg width="500" height="300"></svg> |
||||||
|
<br> |
||||||
|
<input |
||||||
|
type="range" |
||||||
|
v-model="circleSize" |
||||||
|
min="1" |
||||||
|
max="100" |
||||||
|
step="1" |
||||||
|
> |
||||||
|
</div> |
||||||
|
</template> |
||||||
|
|
||||||
|
<script> |
||||||
|
const d3 = require('d3'); |
||||||
|
export default { |
||||||
|
data: function() { |
||||||
|
return { |
||||||
|
circleSize: 50 |
||||||
|
} |
||||||
|
}, |
||||||
|
mounted: function(createElement) { |
||||||
|
var svg = d3.select(this.$el).select('svg'); |
||||||
|
this.circle = svg |
||||||
|
.append('circle') |
||||||
|
.attr('cx', '250') |
||||||
|
.attr('cy', '150') |
||||||
|
.attr('r', this.circleSize) |
||||||
|
}, |
||||||
|
watch: { |
||||||
|
circleSize: function(newValue) { |
||||||
|
this.circle |
||||||
|
.attr('r', newValue) |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
} |
||||||
|
</script> |
||||||
|
|
||||||
|
<style> |
||||||
|
</style> |
Loading…
Reference in new issue