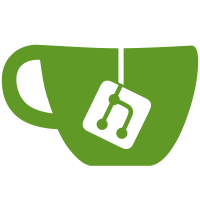
3 changed files with 64 additions and 29 deletions
@ -1,26 +1,49 @@
|
||||
use std::ptr::NonNull; |
||||
|
||||
use async_trait::async_trait; |
||||
use hyper::{Body, Client, Method, Request, StatusCode}; |
||||
|
||||
use crate::{ |
||||
metric::{Metric, MetricResult}, |
||||
types, |
||||
}; |
||||
|
||||
use bytes::Buf as _; |
||||
|
||||
struct Prometheus { |
||||
|
||||
url: String, |
||||
query: String, |
||||
} |
||||
|
||||
impl Prometheus { |
||||
pub fn new() -> Prometheus { |
||||
Prometheus{} |
||||
pub fn new(url: &String, query: &String) -> Prometheus { |
||||
Prometheus { |
||||
url: url.to_owned(), |
||||
query: query.to_owned(), |
||||
} |
||||
} |
||||
|
||||
// TODO: move to utils
|
||||
async fn get(&self, suburl: &str) -> types::Result<(StatusCode, serde_json::Value)> { |
||||
let req = Request::builder() |
||||
.method(Method::GET) |
||||
.uri(self.url.to_owned() + suburl) |
||||
.header("Accept", "application/json") |
||||
.body(Body::empty()) |
||||
.unwrap(); |
||||
|
||||
let client = Client::new(); |
||||
let res = client.request(req).await?; |
||||
let status = res.status(); |
||||
|
||||
let body = hyper::body::aggregate(res).await?; |
||||
let reader = body.reader(); |
||||
let result: serde_json::Value = serde_json::from_reader(reader)?; |
||||
Ok((status, result)) |
||||
} |
||||
} |
||||
|
||||
#[async_trait] |
||||
impl Metric for Prometheus { |
||||
async fn query_chunk(&self, from: u64, to: u64, step: u64) -> types::Result<MetricResult> { |
||||
return None; |
||||
return Ok(Default::default()); |
||||
} |
||||
} |
||||
} |
||||
|
Loading…
Reference in new issue