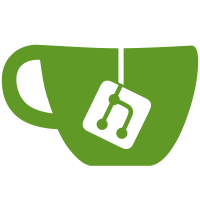
4 changed files with 109 additions and 95 deletions
@ -1,65 +1,72 @@
|
||||
import { runPredict } from './analytics_controller'; |
||||
import { getLabeledSegments } from './segments_controller'; |
||||
import { AnalyticUnitId } from '../models/analytic_unit'; |
||||
import { sendNotification } from '../services/notification_service'; |
||||
import { getJsonDataSync, writeJsonDataSync } from '../services/json_service'; |
||||
import { ANALYTIC_UNITS_PATH } from '../config'; |
||||
|
||||
import * as path from 'path'; |
||||
import * as fs from 'fs'; |
||||
|
||||
|
||||
const ALERT_TIMEOUT = 60000; // ms
|
||||
const ALERTS_DB_PATH = path.join(ANALYTIC_UNITS_PATH, `alerts_anomalies.json`); |
||||
|
||||
|
||||
export function getAlertsAnomalies(): AnalyticUnitId[] { |
||||
if(!fs.existsSync(ALERTS_DB_PATH)) { |
||||
saveAlertsAnomalies([]); |
||||
} |
||||
return getJsonDataSync(ALERTS_DB_PATH); |
||||
} |
||||
|
||||
export function saveAlertsAnomalies(units: AnalyticUnitId[]) { |
||||
return writeJsonDataSync(ALERTS_DB_PATH, units); |
||||
} |
||||
|
||||
function processAlerts(id: AnalyticUnitId) { |
||||
let segments = getLabeledSegments(id); |
||||
|
||||
const currentTime = new Date().getTime(); |
||||
const activeAlert = activeAlerts.has(id); |
||||
let newActiveAlert = false; |
||||
|
||||
if(segments.length > 0) { |
||||
let lastSegment = segments[segments.length - 1]; |
||||
if(lastSegment.finish >= currentTime - ALERT_TIMEOUT) { |
||||
newActiveAlert = true; |
||||
} |
||||
} |
||||
|
||||
if(!activeAlert && newActiveAlert) { |
||||
activeAlerts.add(id); |
||||
sendNotification(id, true); |
||||
} else if(activeAlert && !newActiveAlert) { |
||||
activeAlerts.delete(id); |
||||
sendNotification(id, false); |
||||
} |
||||
} |
||||
|
||||
async function alertsTick() { |
||||
let alertsAnomalies = getAlertsAnomalies(); |
||||
for (let predictorId of alertsAnomalies) { |
||||
try { |
||||
await runPredict(predictorId); |
||||
processAlerts(predictorId); |
||||
} catch (e) { |
||||
console.error(e); |
||||
} |
||||
} |
||||
setTimeout(alertsTick, 5000); |
||||
} |
||||
|
||||
|
||||
const activeAlerts = new Set<string>(); |
||||
setTimeout(alertsTick, 5000); |
||||
/** |
||||
* Alarting is not supported yet |
||||
*/ |
||||
|
||||
throw new console.error("Not supported"); |
||||
|
||||
|
||||
// import { runPredict } from './analytics_controller';
|
||||
// import { getLabeledSegments } from './segments_controller';
|
||||
// import { AnalyticUnitId } from '../models/analytic_unit';
|
||||
// import { sendNotification } from '../services/notification_service';
|
||||
// import { getJsonDataSync, writeJsonDataSync } from '../services/json_service';
|
||||
// import { ANALYTIC_UNITS_PATH } from '../config';
|
||||
|
||||
// import * as path from 'path';
|
||||
// import * as fs from 'fs';
|
||||
|
||||
|
||||
// const ALERT_TIMEOUT = 60000; // ms
|
||||
// const ALERTS_DB_PATH = path.join(ANALYTIC_UNITS_PATH, `alerts_anomalies.json`);
|
||||
|
||||
|
||||
// export function getAlertsAnomalies(): AnalyticUnitId[] {
|
||||
// if(!fs.existsSync(ALERTS_DB_PATH)) {
|
||||
// saveAlertsAnomalies([]);
|
||||
// }
|
||||
// return getJsonDataSync(ALERTS_DB_PATH);
|
||||
// }
|
||||
|
||||
// export function saveAlertsAnomalies(units: AnalyticUnitId[]) {
|
||||
// return writeJsonDataSync(ALERTS_DB_PATH, units);
|
||||
// }
|
||||
|
||||
// function processAlerts(id: AnalyticUnitId) {
|
||||
// let segments = getLabeledSegments(id);
|
||||
|
||||
// const currentTime = new Date().getTime();
|
||||
// const activeAlert = activeAlerts.has(id);
|
||||
// let newActiveAlert = false;
|
||||
|
||||
// if(segments.length > 0) {
|
||||
// let lastSegment = segments[segments.length - 1];
|
||||
// if(lastSegment.finish >= currentTime - ALERT_TIMEOUT) {
|
||||
// newActiveAlert = true;
|
||||
// }
|
||||
// }
|
||||
|
||||
// if(!activeAlert && newActiveAlert) {
|
||||
// activeAlerts.add(id);
|
||||
// sendNotification(id, true);
|
||||
// } else if(activeAlert && !newActiveAlert) {
|
||||
// activeAlerts.delete(id);
|
||||
// sendNotification(id, false);
|
||||
// }
|
||||
// }
|
||||
|
||||
// async function alertsTick() {
|
||||
// let alertsAnomalies = getAlertsAnomalies();
|
||||
// for (let predictorId of alertsAnomalies) {
|
||||
// try {
|
||||
// await runPredict(predictorId);
|
||||
// processAlerts(predictorId);
|
||||
// } catch (e) {
|
||||
// console.error(e);
|
||||
// }
|
||||
// }
|
||||
// setTimeout(alertsTick, 5000);
|
||||
// }
|
||||
|
||||
|
||||
// const activeAlerts = new Set<string>();
|
||||
// setTimeout(alertsTick, 5000);
|
||||
|
@ -1,36 +1,43 @@
|
||||
import * as AnalyticUnit from '../models/analytic_unit'; |
||||
import { getAlertsAnomalies, saveAlertsAnomalies } from '../controllers/alerts_controller'; |
||||
// /**
|
||||
// * Alarting is not supported yet
|
||||
// */
|
||||
|
||||
import * as Router from 'koa-router'; |
||||
// throw new console.error("Not supported");
|
||||
|
||||
|
||||
function getAlert(ctx: Router.IRouterContext) { |
||||
let id: AnalyticUnit.AnalyticUnitId = ctx.request.query.id; |
||||
// import * as AnalyticUnit from '../models/analytic_unit';
|
||||
// import { getAlertsAnomalies, saveAlertsAnomalies } from '../controllers/alerts_controller';
|
||||
|
||||
let alertsAnomalies = getAlertsAnomalies(); |
||||
let pos = alertsAnomalies.indexOf(id); |
||||
// import * as Router from 'koa-router';
|
||||
|
||||
let enabled: boolean = (pos !== -1); |
||||
ctx.response.body = { enabled }; |
||||
} |
||||
|
||||
function setAlertEnabled(ctx: Router.IRouterContext) { |
||||
let id: AnalyticUnit.AnalyticUnitId = ctx.request.body.id; |
||||
let enabled: boolean = ctx.request.body.enabled; |
||||
// function getAlert(ctx: Router.IRouterContext) {
|
||||
// let id: AnalyticUnit.AnalyticUnitId = ctx.request.query.id;
|
||||
|
||||
let alertsAnomalies = getAlertsAnomalies(); |
||||
let pos: number = alertsAnomalies.indexOf(id); |
||||
if(enabled && pos == -1) { |
||||
alertsAnomalies.push(id); |
||||
saveAlertsAnomalies(alertsAnomalies); |
||||
} else if(!enabled && pos > -1) { |
||||
alertsAnomalies.splice(pos, 1); |
||||
saveAlertsAnomalies(alertsAnomalies); |
||||
} |
||||
ctx.response.body = { status: 'OK' }; |
||||
} |
||||
// let alertsAnomalies = getAlertsAnomalies();
|
||||
// let pos = alertsAnomalies.indexOf(id);
|
||||
|
||||
export const router = new Router(); |
||||
// let enabled: boolean = (pos !== -1);
|
||||
// ctx.response.body = { enabled };
|
||||
// }
|
||||
|
||||
router.get('/', getAlert); |
||||
router.post('/', setAlertEnabled); |
||||
// function setAlertEnabled(ctx: Router.IRouterContext) {
|
||||
// let id: AnalyticUnit.AnalyticUnitId = ctx.request.body.id;
|
||||
// let enabled: boolean = ctx.request.body.enabled;
|
||||
|
||||
// let alertsAnomalies = getAlertsAnomalies();
|
||||
// let pos: number = alertsAnomalies.indexOf(id);
|
||||
// if(enabled && pos == -1) {
|
||||
// alertsAnomalies.push(id);
|
||||
// saveAlertsAnomalies(alertsAnomalies);
|
||||
// } else if(!enabled && pos > -1) {
|
||||
// alertsAnomalies.splice(pos, 1);
|
||||
// saveAlertsAnomalies(alertsAnomalies);
|
||||
// }
|
||||
// ctx.response.body = { status: 'OK' };
|
||||
// }
|
||||
|
||||
// export const router = new Router();
|
||||
|
||||
// router.get('/', getAlert);
|
||||
// router.post('/', setAlertEnabled);
|
||||
|
Loading…
Reference in new issue