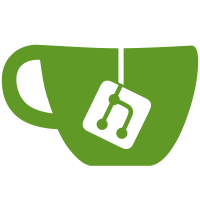
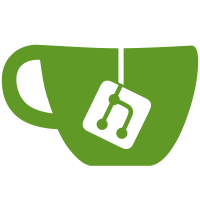
11 changed files with 135 additions and 6 deletions
@ -0,0 +1,18 @@
|
||||
import { exportPanel } from '../services/export_service'; |
||||
|
||||
import * as Router from 'koa-router'; |
||||
|
||||
|
||||
async function getPanelTemplate(ctx: Router.IRouterContext) { |
||||
let panelId = ctx.request.query.panelId; |
||||
if(panelId === undefined) { |
||||
throw new Error('Cannot export analytic units with undefined panelId'); |
||||
} |
||||
|
||||
const json = await exportPanel(panelId); |
||||
ctx.response.body = json; |
||||
} |
||||
|
||||
export var router = new Router(); |
||||
|
||||
router.get('/template', getPanelTemplate); |
@ -0,0 +1,29 @@
|
||||
import * as AnalyticUnit from '../models/analytic_units'; |
||||
import * as AnalyticUnitCache from '../models/analytic_unit_cache_model'; |
||||
import * as DetectionSpan from '../models/detection_model'; |
||||
import * as Segment from '../models/segment_model'; |
||||
|
||||
|
||||
export async function exportPanel(panelId: string): Promise<{ |
||||
analyticUnitTemplates: any[], |
||||
caches: AnalyticUnitCache.AnalyticUnitCache[], |
||||
detectionSpans: DetectionSpan.DetectionSpan[], |
||||
segments: Segment.Segment[] |
||||
}> { |
||||
const analyticUnits = await AnalyticUnit.findMany({ panelId }); |
||||
const analyticUnitIds = analyticUnits.map(analyticUnit => analyticUnit.id); |
||||
const analyticUnitTemplates = analyticUnits.map(analyticUnit => analyticUnit.toTemplate()); |
||||
|
||||
const [caches, detectionSpans, segments] = await Promise.all([ |
||||
AnalyticUnitCache.findMany({ _id: { $in: analyticUnitIds } }), |
||||
DetectionSpan.findByAnalyticUnitIds(analyticUnitIds), |
||||
Segment.findByAnalyticUnitIds(analyticUnitIds) |
||||
]); |
||||
|
||||
return { |
||||
analyticUnitTemplates, |
||||
caches, |
||||
detectionSpans, |
||||
segments |
||||
}; |
||||
} |
@ -0,0 +1,7 @@
|
||||
// We should remove Omit definition when we'll be updating TypeScript
|
||||
// It was introduced in TS v3.5.1:
|
||||
// https://devblogs.microsoft.com/typescript/announcing-typescript-3-5/#the-omit-helper-type
|
||||
/** |
||||
* Construct a type with the properties of T except for those in type K. |
||||
*/ |
||||
export type Omit<T, K extends string | number | symbol> = { [P in Exclude<keyof T, K>]: T[P]; } |
Loading…
Reference in new issue