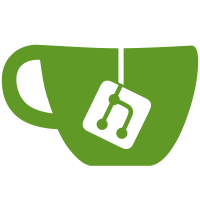
12 changed files with 241 additions and 232 deletions
@ -1,30 +0,0 @@
|
||||
import { getJsonDataSync, writeJsonDataSync } from '../services/json_service'; |
||||
import { METRICS_PATH } from '../config'; |
||||
|
||||
import * as crypto from 'crypto'; |
||||
|
||||
import * as path from 'path'; |
||||
|
||||
|
||||
function saveTargets(targets) { |
||||
let metrics = []; |
||||
for (let target of targets) { |
||||
metrics.push(saveTarget(target)); |
||||
} |
||||
return metrics; |
||||
} |
||||
|
||||
function saveTarget(target) { |
||||
//const md5 = crypto.createHash('md5')
|
||||
const targetId = crypto.createHash('md5').update(JSON.stringify(target)).digest('hex'); |
||||
let filename = path.join(METRICS_PATH, `${targetId}.json`); |
||||
writeJsonDataSync(filename, target); |
||||
return targetId; |
||||
} |
||||
|
||||
function getTarget(targetId) { |
||||
let filename = path.join(METRICS_PATH, `${targetId}.json`); |
||||
return getJsonDataSync(filename); |
||||
} |
||||
|
||||
export { saveTargets, getTarget } |
@ -1,84 +0,0 @@
|
||||
import { loadFile, saveFile } from '../services/data_service'; |
||||
|
||||
import * as crypto from 'crypto'; |
||||
|
||||
import * as path from 'path' |
||||
import * as fs from 'fs' |
||||
|
||||
|
||||
export type Datasource = { |
||||
method: string, |
||||
data: Object, |
||||
params: Object, |
||||
type: string, |
||||
url: string |
||||
} |
||||
|
||||
export type Metric = { |
||||
datasource: string, |
||||
targets: string[] |
||||
} |
||||
|
||||
export type AnalyticUnitId = string; |
||||
|
||||
export type AnalyticUnit = { |
||||
id?: AnalyticUnitId, |
||||
name: string, |
||||
panelUrl: string, |
||||
type: string, |
||||
metric: Metric, |
||||
datasource: Datasource |
||||
status: string, |
||||
error?: string, |
||||
lastPredictionTime: number, |
||||
nextId: number |
||||
} |
||||
|
||||
export function createItem(item: AnalyticUnit): AnalyticUnitId { |
||||
const hashString = item.name + (new Date()).toString(); |
||||
const newId: AnalyticUnitId = crypto.createHash('md5').update(hashString).digest('hex'); |
||||
let filename = `${newId}.json`; |
||||
if(fs.existsSync(filename)) { |
||||
throw new Error(`Can't create item with id ${newId}`); |
||||
} |
||||
save(newId, item); |
||||
item.id = newId; |
||||
return newId; |
||||
} |
||||
|
||||
export function remove(id: AnalyticUnitId) { |
||||
let filename = `${id}.json`; |
||||
fs.unlinkSync(filename); |
||||
} |
||||
|
||||
export function save(id: AnalyticUnitId, unit: AnalyticUnit) { |
||||
let filename = `${id}.json`; |
||||
return saveFile(filename, JSON.stringify(unit)); |
||||
} |
||||
|
||||
// TODO: make async
|
||||
export async function findById(id: AnalyticUnitId): Promise<AnalyticUnit> { |
||||
let filename = `${id}.json`; |
||||
if(!fs.existsSync(filename)) { |
||||
throw new Error(`Can't find Analytic Unit with id ${id}`); |
||||
} |
||||
let result = await loadFile(filename); |
||||
return JSON.parse(result); |
||||
} |
||||
|
||||
export async function setStatus(predictorId: AnalyticUnitId, status: string, error?: string) { |
||||
let info = await findById(predictorId); |
||||
info.status = status; |
||||
if(error !== undefined) { |
||||
info.error = error; |
||||
} else { |
||||
info.error = ''; |
||||
} |
||||
save(predictorId, info); |
||||
} |
||||
|
||||
export async function setPredictionTime(id: AnalyticUnitId, time: number) { |
||||
let info = await findById(id); |
||||
info.lastPredictionTime = time; |
||||
save(id, info); |
||||
} |
@ -0,0 +1,84 @@
|
||||
import { Metric, metricFromObj } from './metric_model'; |
||||
import { Collection, makeDBQ } from '../services/data_service'; |
||||
|
||||
|
||||
let db = makeDBQ(Collection.ANALYTIC_UNITS); |
||||
|
||||
|
||||
|
||||
export type AnalyticUnitId = string; |
||||
|
||||
export type AnalyticUnit = { |
||||
id?: AnalyticUnitId, |
||||
name: string, |
||||
panelUrl: string, |
||||
type: string, |
||||
metric: Metric |
||||
status: string, |
||||
error?: string, |
||||
lastPredictionTime: number, |
||||
nextId: number |
||||
} |
||||
|
||||
|
||||
export function analyticUnitFromObj(obj: any): AnalyticUnit { |
||||
if(obj === undefined) { |
||||
throw new Error('obj is undefined'); |
||||
} |
||||
if(obj.type === undefined) { |
||||
throw new Error(`Missing field "type"`); |
||||
} |
||||
if(obj.name === undefined) { |
||||
throw new Error(`Missing field "name"`); |
||||
} |
||||
if(obj.panelUrl === undefined) { |
||||
throw new Error(`Missing field "panelUrl"`); |
||||
} |
||||
if(obj.metric === undefined) { |
||||
throw new Error(`Missing field "datasource"`); |
||||
} |
||||
if(obj.metric.datasource === undefined) { |
||||
throw new Error(`Missing field "metric.datasource"`); |
||||
} |
||||
if(obj.metric.targets === undefined) { |
||||
throw new Error(`Missing field "metric.targets"`); |
||||
} |
||||
|
||||
const unit: AnalyticUnit = { |
||||
name: obj.name, |
||||
panelUrl: obj.panelUrl, |
||||
type: obj.type, |
||||
datasource: obj.datasource, |
||||
metric: metric, |
||||
status: 'LEARNING', |
||||
lastPredictionTime: 0, |
||||
nextId: 0 |
||||
}; |
||||
|
||||
return unit; |
||||
} |
||||
|
||||
export async function findById(id: AnalyticUnitId): Promise<AnalyticUnit> { |
||||
return db.findOne(id); |
||||
} |
||||
|
||||
export async function create(unit: AnalyticUnit): Promise<AnalyticUnitId> { |
||||
return db.insert(unit); |
||||
} |
||||
|
||||
export async function remove(id: AnalyticUnitId): Promise<void> { |
||||
await db.remove(id); |
||||
return; |
||||
} |
||||
|
||||
export async function update(id: AnalyticUnitId, unit: AnalyticUnit) { |
||||
return db.update(id, unit); |
||||
} |
||||
|
||||
export async function setStatus(id: AnalyticUnitId, status: string, error?: string) { |
||||
return db.update(id, { status, error }); |
||||
} |
||||
|
||||
export async function setPredictionTime(id: AnalyticUnitId, lastPredictionTime: number) { |
||||
return db.update(id, { lastPredictionTime }); |
||||
} |
@ -0,0 +1,49 @@
|
||||
import { Collection, makeDBQ } from '../services/data_service'; |
||||
|
||||
|
||||
let db = makeDBQ(Collection.METRICS); |
||||
|
||||
|
||||
export type Datasource = { |
||||
method: string, |
||||
data: Object, |
||||
params: Object, |
||||
type: string, |
||||
url: string |
||||
} |
||||
|
||||
export type Metric = { |
||||
datasource: Datasource, |
||||
targets: string[] |
||||
} |
||||
|
||||
export function metricFromObj(obj: any): Metric { |
||||
const metric: Metric = { |
||||
datasource: obj.datasource, |
||||
targets: obj.targets; |
||||
}; |
||||
return metric; |
||||
} |
||||
|
||||
export async function saveTargets(targets: string[]) { |
||||
let metrics = []; |
||||
for (let target of targets) { |
||||
metrics.push(saveTarget(target)); |
||||
} |
||||
return metrics; |
||||
} |
||||
|
||||
export async function saveTarget(target: string) { |
||||
//const md5 = crypto.createHash('md5')
|
||||
const targetId = crypto.createHash('md5').update(JSON.stringify(target)).digest('hex'); |
||||
let filename = path.join(METRICS_PATH, `${targetId}.json`); |
||||
writeJsonDataSync(filename, target); |
||||
return targetId; |
||||
} |
||||
|
||||
export async function getTarget(targetId) { |
||||
let filename = path.join(METRICS_PATH, `${targetId}.json`); |
||||
return getJsonDataSync(filename); |
||||
} |
||||
|
||||
|
Loading…
Reference in new issue