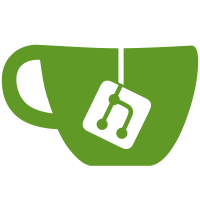
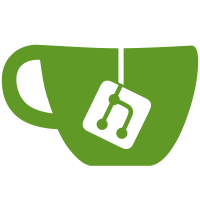
4 changed files with 115 additions and 0 deletions
@ -0,0 +1,75 @@
|
||||
import { TEST_ANALYTIC_UNIT_ID } from '../utils_for_tests/analytic_units'; |
||||
import { buildSpans, insertSpans, clearSpansDB, convertSpansToOptions } from '../utils_for_tests/detection_spans'; |
||||
|
||||
import * as Detection from '../../src/models/detection_model'; |
||||
|
||||
import * as _ from 'lodash'; |
||||
|
||||
const INITIAL_SPANS_CONFIGS = [ |
||||
{ from: 1, to: 3, status: Detection.DetectionStatus.READY }, |
||||
{ from: 4, to: 5, status: Detection.DetectionStatus.RUNNING } |
||||
]; |
||||
|
||||
beforeEach(async () => { |
||||
await insertSpans(INITIAL_SPANS_CONFIGS); |
||||
}); |
||||
|
||||
afterEach(clearSpansDB); |
||||
|
||||
describe('insertSpan', () => { |
||||
it('should merge spans with the same status', async () => { |
||||
await insertSpans([ |
||||
{ from: 3, to: 5, status: Detection.DetectionStatus.READY } |
||||
]); |
||||
|
||||
const expectedSpans = [ |
||||
{ from: 1, to: 5, status: Detection.DetectionStatus.READY } |
||||
]; |
||||
const spansInDB = await Detection.findMany(TEST_ANALYTIC_UNIT_ID, { }); |
||||
const spansOptions = convertSpansToOptions(spansInDB); |
||||
expect(spansOptions).toEqual(expectedSpans); |
||||
}); |
||||
|
||||
|
||||
it('should merge spans if existing span is inside the one being inserted', async () => { |
||||
await insertSpans([ |
||||
{ from: 1, to: 6, status: Detection.DetectionStatus.RUNNING } |
||||
]); |
||||
|
||||
const expectedSpans = [ |
||||
{ from: 1, to: 6, status: Detection.DetectionStatus.RUNNING } |
||||
]; |
||||
const spansInDB = await Detection.findMany(TEST_ANALYTIC_UNIT_ID, {}); |
||||
const spansOptions = convertSpansToOptions(spansInDB); |
||||
expect(spansOptions).toEqual(expectedSpans); |
||||
}); |
||||
}); |
||||
|
||||
describe('getIntersectedSpans', () => { |
||||
it('should find all intersections with the inserted span', async () => { |
||||
const testCases = [ |
||||
{ |
||||
from: 1, to: 5, |
||||
expected: [ |
||||
{ from: 1, to: 3, status: Detection.DetectionStatus.READY }, |
||||
{ from: 3, to: 4, status: Detection.DetectionStatus.RUNNING } |
||||
] |
||||
}, |
||||
{ from: 4, to: 5, expected: [{ from: 3, to: 4, status: Detection.DetectionStatus.RUNNING }] }, |
||||
{ from: 6, to: 7, expected: [] } |
||||
] |
||||
|
||||
for(let testCase of testCases) { |
||||
const intersectedSpans = await Detection.getIntersectedSpans(TEST_ANALYTIC_UNIT_ID, testCase.from, testCase.to); |
||||
const intersectedSpansOptions = convertSpansToOptions(intersectedSpans); |
||||
expect(intersectedSpansOptions).toEqual(testCase.expected); |
||||
} |
||||
}); |
||||
}); |
||||
|
||||
describe('getSpanBorders', () => { |
||||
it('should sort and find span borders', () => { |
||||
const borders = Detection.getSpanBorders(buildSpans(INITIAL_SPANS_CONFIGS)); |
||||
expect(borders).toEqual([1, 3, 3, 4]); |
||||
}); |
||||
}); |
@ -0,0 +1,28 @@
|
||||
import { TEST_ANALYTIC_UNIT_ID } from './analytic_units'; |
||||
|
||||
import * as Detection from '../../src/models/detection_model'; |
||||
|
||||
import * as _ from 'lodash'; |
||||
|
||||
export type DetectionSpanOptions = { from: number, to: number, status: Detection.DetectionStatus }; |
||||
|
||||
export function buildSpans(options: DetectionSpanOptions[]): Detection.DetectionSpan[] { |
||||
return options.map(option => { |
||||
return new Detection.DetectionSpan(TEST_ANALYTIC_UNIT_ID, option.from, option.to, option.status); |
||||
}); |
||||
} |
||||
|
||||
export async function insertSpans(options: DetectionSpanOptions[]): Promise<void> { |
||||
const spansToInsert = buildSpans(options); |
||||
const insertPromises = spansToInsert.map(async span => Detection.insertSpan(span)); |
||||
await Promise.all(insertPromises); |
||||
} |
||||
|
||||
export function convertSpansToOptions(spans: Detection.DetectionSpan[]): DetectionSpanOptions[] { |
||||
const spansOptions = spans.map(span => ({ from: span.from, to: span.to, status: span.status })); |
||||
return _.sortBy(spansOptions, spanOptions => spanOptions.from); |
||||
} |
||||
|
||||
export async function clearSpansDB(): Promise<void> { |
||||
await Detection.clearSpans(TEST_ANALYTIC_UNIT_ID); |
||||
} |
Loading…
Reference in new issue