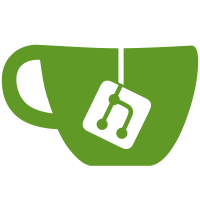
1 changed files with 62 additions and 0 deletions
@ -0,0 +1,62 @@ |
|||||||
|
use std::collections::HashSet; |
||||||
|
|
||||||
|
struct PN { |
||||||
|
a: u32, |
||||||
|
b: u32, |
||||||
|
ps: String, |
||||||
|
} |
||||||
|
|
||||||
|
impl PN { |
||||||
|
fn new(a: u32, b: u32) -> PN { |
||||||
|
PN { a, b, ps: String::new() } |
||||||
|
} |
||||||
|
|
||||||
|
fn simplify(&mut self, ps: &Vec<u32> ) { |
||||||
|
let mut n = self.a; |
||||||
|
for p in ps { |
||||||
|
let mut k = 0; |
||||||
|
while n % p == 0 { k += 1; n /= p; } |
||||||
|
k *= self.b; |
||||||
|
if k > 0 { |
||||||
|
self.ps += &format!("{},{}.", p, k); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
fn er() -> Vec<u32> { |
||||||
|
let mut ns = Vec::new(); |
||||||
|
let mut res = Vec::new(); |
||||||
|
for _i in 0..=100 { ns.push(true); } |
||||||
|
let mut k = 1; |
||||||
|
loop { |
||||||
|
let mut found_next_k = false; |
||||||
|
for j in k + 1..=100 { if ns[j] { k = j; found_next_k = true; break; } } |
||||||
|
if !found_next_k { break; } |
||||||
|
res.push(k as u32); |
||||||
|
for i in (k+k..=100).step_by(k) { ns[i] = false } |
||||||
|
} |
||||||
|
|
||||||
|
return res; |
||||||
|
} |
||||||
|
|
||||||
|
fn main() { |
||||||
|
let mut hs = HashSet::new(); |
||||||
|
let ps = er(); |
||||||
|
for p in &ps { print!("{} ", p); } |
||||||
|
println!(""); |
||||||
|
|
||||||
|
for a in 2..=100 { |
||||||
|
for b in 2..=100 { |
||||||
|
let mut pn = PN::new(a, b); |
||||||
|
pn.simplify(&ps); |
||||||
|
//println!("{}", pn.ps);
|
||||||
|
hs.insert(pn.ps); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
println!("{}", hs.len()); |
||||||
|
|
||||||
|
|
||||||
|
} |
||||||
|
|
Loading…
Reference in new issue